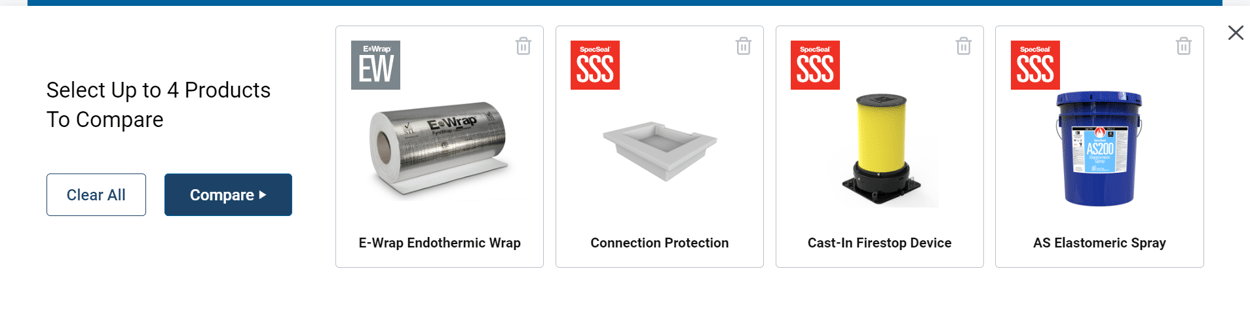
JS for compare listing page products in compare list via cookie.
<div class="compare_popup_wrapper">
<div class="container">
<div class="row align-items-center justify-content-between">
<div class="compare_popup_button col-sm-3">
<h4>
Select Up to 4 Products <br>To Compare
</h4>
<button class="button pop_trans_btn" id="clear-all-button" onclick="clearAll()">Clear All</button>
<a class="button pop_blue_btn" href="javascript:void(0);" onclick="openComparisonPage()">Compare
<img src="https://44097813.fs1.hubspotusercontent-na1.net/hubfs/44097813/STI_hs_files/right-arrow.svg"
alt="arrow" />
</a>
<div id="pop_max_prod_error" class="mt-4" style="color: red;"></div>
</div>
<div class="compare_popup_content col-sm-9">
<div id="popup-container">
<!-- Initially hidden -->
</div>
</div>
</div>
</div>
</div>
<script>
// Function to clear all products from the comparison list
function clearAll() {
setComparisonList([]); // Set an empty array to clear the comparison list
updateCheckboxes();
showSelectedProducts();
}
// Function to add or remove a product from the comparison list
function handleCheckboxChange(checkbox) {
var comparisonList = getComparisonList();
var maxProducts = 4;
var errorContainer = document.getElementById('pop_max_prod_error');
var productItem = checkbox.closest('.product-items');
var productId = productItem.getAttribute('data-product-id');
var productName = productItem.querySelector('.product-name').textContent;
var brandLogoContainer = productItem.querySelector('.brand-logo');
var brandLogoImg = brandLogoContainer ? brandLogoContainer.querySelector('div') : null;
var brandLogoClass = brandLogoImg ? brandLogoImg.getAttribute('class') : '';
var productImage = productItem.querySelector('.product-image img').getAttribute('src');
var prod_link = productItem.querySelector('.prod_link').textContent;
if (checkbox.checked) {
if (comparisonList.length < maxProducts) {
displayCookieValue();
// Add the product to the comparison list
addToComparisonList(
productId,
productName,
brandLogoClass,
productImage
);
errorContainer.textContent = ''; // Clear any codevious error messages
} else {
// If the limit is reached, display an error message
checkbox.checked = false;
errorContainer.textContent = 'Maximum 4 products can be added';
}
} else {
// Remove the product from the comparison list
removeFromComparisonList(productId);
errorContainer.textContent = ''; // Clear any codevious error messages
}
showSelectedProducts();
displayCookieValue();
}
// Function to update the Compare button based on the comparison list count
function updateCompareButton() {
var comparisonList = getComparisonList();
var compareButton = document.querySelector('.button.pop_blue_btn');
if (comparisonList.length >= 2) {
compareButton.classList.remove('disabled');
} else {
compareButton.classList.add('disabled');
}
}
// Function to add a product to the comparison list
function addToComparisonList(
productId,
productName,
brandLogoClass,
productImage
) {
var comparisonList = getComparisonList();
//var encodedCertifications = btoa(Certifications);
//var encodedApplications = btoa(Applications);
// Check if the product is not already in the list to avoid duplicates
if (!comparisonList.some(item => item.id === productId)) {
comparisonList.push({
id:productId,
name:productName,
brandLogo:brandLogoClass,
productImage:productImage
});
}
setComparisonList(comparisonList);
updateCompareButton();
}
// Function to remove a product from the comparison list
function removeFromComparisonList(productId) {
var comparisonList = getComparisonList();
var index = comparisonList.findIndex(item => item.id === productId);
if (index !== -1) {
comparisonList.splice(index, 1);
}
setComparisonList(comparisonList);
updateCompareButton();
}
// Function to get the comparison list from the cookie
function getComparisonList() {
var cookieValue = document.cookie.replace(/(?:(?:^|.*;\s*)comparisonList\s*\=\s*([^;]*).*$)|^.*$/, "$1");
return cookieValue ? JSON.parse(cookieValue) : [];
}
// Function to set the comparison list in the cookie
function setComparisonList(comparisonList) {
document.cookie = 'comparisonList=' + JSON.stringify(comparisonList) + '; path=/';
}
function displayCookieValue() {
const cookieValue = document.cookie.replace(/(?:(?:^|.*;\s*)comparisonList\s*\=\s*([^;]*).*$)|^.*$/, '$1');
console.log(cookieValue);
}
// Call the function to display the cookie value in the console
displayCookieValue();
// Function to display selected products in the popup container
function showSelectedProducts() {
var comparisonList = getComparisonList();
var popupContainer = document.getElementById('popup-container');
var popupWrapper = document.querySelector('.compare_popup_wrapper'); // Get the wrapper element
// Clear the container
popupContainer.innerHTML = '';
var maxProducts = 4;
var placeholdersNeeded = maxProducts - comparisonList.length;
// Display placeholders for remaining slots inside the ul element
var ul = document.createElement('ul');
for (var i = 0; i < placeholdersNeeded; i++) {
var placeholder = document.createElement('li');
placeholder.className = 'placeholder';
ul.appendChild(placeholder);
}
if (comparisonList.length > 0) {
comparisonList.forEach(function (product) {
var li = document.createElement('li');
var brandLogoContainer = document.createElement('div');
brandLogoContainer.className = 'brand-logo-popup';
//var brandLogo = document.createElement('img');
//brandLogo.src = product.brandLogo || ''; // Use empty string if brandLogo is undefined
//brandLogoContainer.appendChild(brandLogo);
// Add span for the class from the img tag
var brandClassSpan = document.createElement('span');
var imgTag = brandLogoContainer.querySelector('div');
var imgClass = imgTag ? imgTag.classList.item(0) : ''; // Get the class from the img tag
brandClassSpan.textContent = imgClass || ''; // Set the class on the span, or an empty string if not found
// Set the class dynamically on the brandClassSpan
brandClassSpan.className = 'brand-class ' + (product.brandLogo || ''); // Add a class to the span
brandLogoContainer.appendChild(brandClassSpan);
var productImageContainer = document.createElement('div');
productImageContainer.className = 'product-image-popup';
var productImage = document.createElement('img');
productImage.src = product.productImage || ''; // Use an empty string if productImage is undefined
productImageContainer.appendChild(productImage);
var productInfo = document.createElement('div');
productInfo.className = 'pop_prod_name';
productInfo.textContent = product.name;
var removeButton = document.createElement('span');
removeButton.className = 'remove-button';
removeButton.textContent = 'Remove';
removeButton.addEventListener('click', function () {
removeFromComparisonList(product.id);
showSelectedProducts();
// Uncheck the corresponding checkbox
var checkbox = document.querySelector('[data-product-id="' + product.id + '"] .compare-checkbox');
if (checkbox) {
checkbox.checked = false;
}
});
li.appendChild(brandLogoContainer);
li.appendChild(productImageContainer);
li.appendChild(productInfo);
li.appendChild(removeButton);
ul.appendChild(li);
});
var closeButton = document.createElement('button');
closeButton.textContent = 'Close';
closeButton.className = 'button pop_trans_btn close-button';
closeButton.addEventListener('click', function () {
popupWrapper.style.display = 'none';
});
popupContainer.appendChild(closeButton);
popupContainer.appendChild(ul);
popupContainer.style.display = 'block'; // Show the container
popupWrapper.style.display = 'block'; // Show the wrapper
} else {
popupContainer.style.display = 'none'; // Hide the container
popupWrapper.style.display = 'none'; // Hide the wrapper
}
}
// Function to update checkboxes based on the comparison list
function updateCheckboxes() {
var checkboxes = document.querySelectorAll('.compare-checkbox');
var comparisonList = getComparisonList();
checkboxes.forEach(function (checkbox) {
checkbox.checked = false; // Uncheck all checkboxes initially
var productId = checkbox.closest('.product-items').getAttribute('data-product-id');
comparisonList.forEach(function (product) {
if (productId === product.id) {
checkbox.checked = true; // Check the checkbox if the product is in the comparison list
}
});
});
}
// Add event listeners to checkboxes
var checkboxes = document.querySelectorAll('.compare-checkbox');
for (var i = 0; i < checkboxes.length; i++) {
checkboxes[i].addEventListener('change', function () {
handleCheckboxChange(this);
});
}
// Function to open the "/comparison" page with the selected products
function openComparisonPage() {
var comparisonList = getComparisonList();
var comparisonParams = comparisonList.map(function (product) {
return 'id=' + product.id;
});
var url = '/comparison?' + comparisonParams.join('&');
window.open(url, '_blank');
}
// Update checkboxes and display selected products on page load
updateCheckboxes();
showSelectedProducts();
// Add an event listener for the DOMContentLoaded event
document.addEventListener('DOMContentLoaded', function () {
// Update checkboxes and display selected products on page load
updateCheckboxes();
showSelectedProducts();
});
</script>
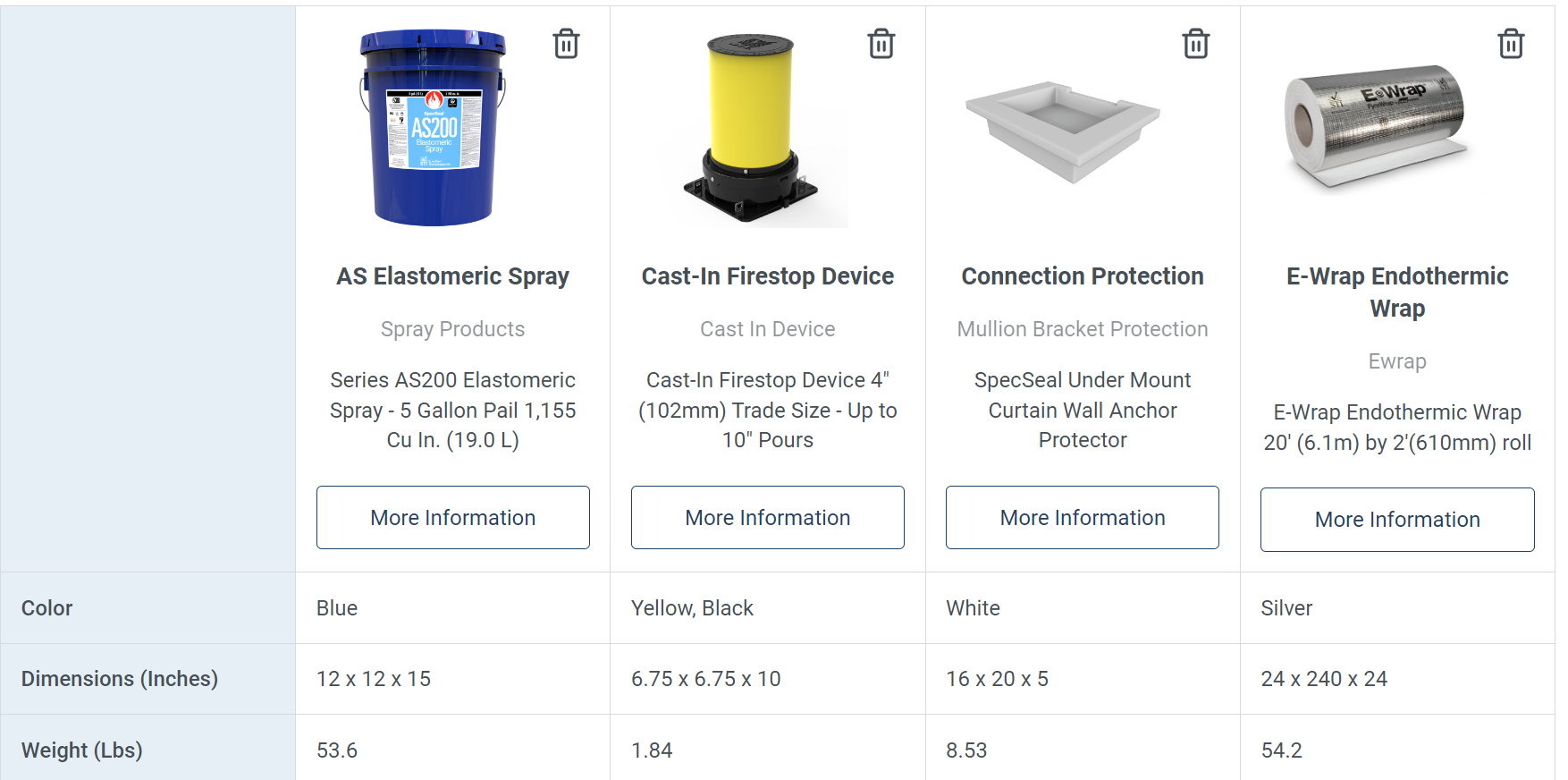
Detail page
<div class="Compare_PLP_STI_23_top_pane pt-4">
<div class="content-wrapper">
<h2 class="m-0">
<strong>Product Comparison</strong>
</h2>
<h5 class="m-0">
Comparing <span>0</span> Products
</h5>
</div>
</div>
<div class="content-wrapper share_wrapper pb-5 pt-3">
<div class="share row align-items-center">
<span class="share_button button grey-button">
<img src="https://staging-www.stifirestop.com/hubfs/Share.svg" alt="Share" loading="lazy" width="18" height="18"
style="max-width: 100%; height: auto;">
Share
</span>
<nav>
<a href="#">
<svg xmlns="http://www.w3.org/2000/svg" height="1em" viewBox="0 0 512 512">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path d="M389.2 48h70.6L305.6 224.2 487 464H345L233.7 318.6 106.5 464H35.8L200.7 275.5 26.8 48H172.4L272.9 180.9 389.2 48zM364.4 421.8h39.1L151.1 88h-42L364.4 421.8z"/>
</svg>
</a>
<a href="#">
<svg xmlns="http://www.w3.org/2000/svg" height="1em" viewBox="0 0 320 512">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path d="M279.14 288l14.22-92.66h-88.91v-60.13c0-25.35 12.42-50.06 52.24-50.06h40.42V6.26S260.43 0 225.36 0c-73.22 0-121.08 44.38-121.08 124.72v70.62H22.89V288h81.39v224h100.17V288z"/>
</svg>
</a>
<a href="#">
<svg xmlns="http://www.w3.org/2000/svg" height="1em" viewBox="0 0 448 512">
<!--! Font Awesome Free 6.4.2 by @fontawesome - https://fontawesome.com License - https://fontawesome.com/license (Commercial License) Copyright 2023 Fonticons, Inc. -->
<path d="M100.28 448H7.4V148.9h92.88zM53.79 108.1C24.09 108.1 0 83.5 0 53.8a53.79 53.79 0 0 1 107.58 0c0 29.7-24.1 54.3-53.79 54.3zM447.9 448h-92.68V302.4c0-34.7-.7-79.2-48.29-79.2-48.29 0-55.69 37.7-55.69 76.7V448h-92.78V148.9h89.08v40.8h1.3c12.4-23.5 42.69-48.3 87.88-48.3 94 0 111.28 61.9 111.28 142.3V448z"/>
</svg>
</a>
</nav>
</div>
</div>
<div class="Compare_PLP_STI_23_main_container row-fluid pb-5 content-wrapper">
<div class="fixedTable row" id="compare_table">
<table id="comparison-table" class="table table-bordered comparison-table">
<tbody>
<tr>
<td> </td>
</tr>
<tr>
<td>Color</td>
</tr>
<tr>
<td>Dimensions (Inches)</td>
</tr>
<tr>
<td>Weight (Lbs)</td>
</tr>
<tr>
<td>Fire Rating</td>
</tr>
<tr>
<td>Special Features</td>
</tr>
<tr class="icons">
<td>Applications</td>
</tr>
<tr class="icons">
<td>Properties</td>
</tr>
<tr class="icons">
<td> </td>
</tr>
</tbody>
</table>
</div>
</div>
read cookie by javascript on page first
document.addEventListener("DOMContentLoaded", (event) => {
$('.remove-action').on('click', (event)=> {
removeItemFromComparisonList(event.currentTarget.dataset.productId);
})
})
function removeItemFromComparisonList(productId) {
let cookieValue = JSON.parse(document.cookie.replace(/(?:(?:^|.*;\s*)comparisonList\s*\=\s*([^;]*).*$)|^.*$/, '$1'));
var index = cookieValue.findIndex(item => item.id === productId);
if (index !== -1) {
cookieValue.splice(index, 1);
}
document.cookie = "comparisonList="+JSON.stringify(cookieValue);
document.location.reload();
}
========================================================
Second approach for detail page
<p>No products selected for comparison.</p>
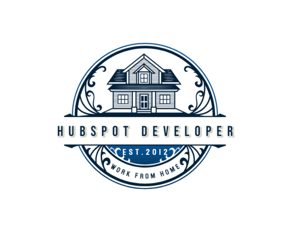